In this tutorial, you will learn how to create your own Gutenberg blocks to be used in App Pages and the App Editor. For more information about how app blocks and the App Editor work, it is explained and demoed in the Blog Posts tutorial (you can fast forward to 2:15 in the video).
We are supporting a number of blocks designed to work natively in the BuddyBoss App, but you may want to create your own blocks for your custom functionality.
Once a block is registered, you’ll need to follow the instructions in our Adding Custom Gutenberg Blocks into your App tutorial to configure how it will render in your app.
You can optionally skip to the “Example Plugin” section at the end of the article, for an example plugin you can quickly test with.
Note: This tutorial requires BuddyBoss App Plugin v1.0.4 or higher.
1. Create one class file and extend ‘BuddyBossApp\Admin\GutenbergBlockAbstract’
Use BuddyBossApp\Admin\GutenbergBlockAbstract; class <ClassName> extends GutenbergBlockAbstract { public function __construct() { } function get_results( $attributes ) { // TODO: Implement get_results() method. } }
2. Once the class is created, set a block argument using the Setter method.
blockNamespace : Block namespace, eg. bbapp/books
blockTitle : Block title.
blockDescription : Block description.
blockIcon : Block Icon. Use Dashicons.
blockKeywords : Block keyword.
attributes : Block attribute, including block setting fields.
preview : Preview HTML for the web when the block is added in the editor or page content.
Use BuddyBossApp\Admin\GutenbergBlockAbstract; class <ClassName> extends GutenbergBlockAbstract { public function __construct() { $this->set_namespace( $blockNamespace ); $this->set_title( $blockTitle ); $this->set_description( $blockDescription ); $this->set_icon( $blockIcon ); $this->set_keywords( $blockKeywords ); $this->set_attributes( $attributes ); $this->set_preview( $preview ); } }
3. Once the attribute is set, execute the parent class init
method, which will add hooks to the registered block.
4. To send block data with the same app page endpoint, use the get_results
method.
function get_results( $attrs ) {
return $data;
}
5. In other cases, you can send a data_source
argument with the app page endpoint, and the app will know that it will need to fetch data before rendering your block.
App_page_data : Block rest response
block_data : Block details.
In data_source
you need to pass two values:
type : Value should be`fetch`
Request_params : Request argument to fetch data
Note: This Request_params
argument is prepared from the WordPress side because doing this in the app would require a new app build every time you change the argument or its value.
function update_block_data( $app_page_data, $block_data ) {
$data_source = array(
'type' => 'fetch',
'request_params' => $request_params,
);
$app_page_data['data']['data_source'] = $data_source;
return $app_page_data;
}
Now, the block should be available when editing content on an App Page. Once a block is used in an App Page, you will get block data similar to the one below, in the endpoint to be handled in the app.
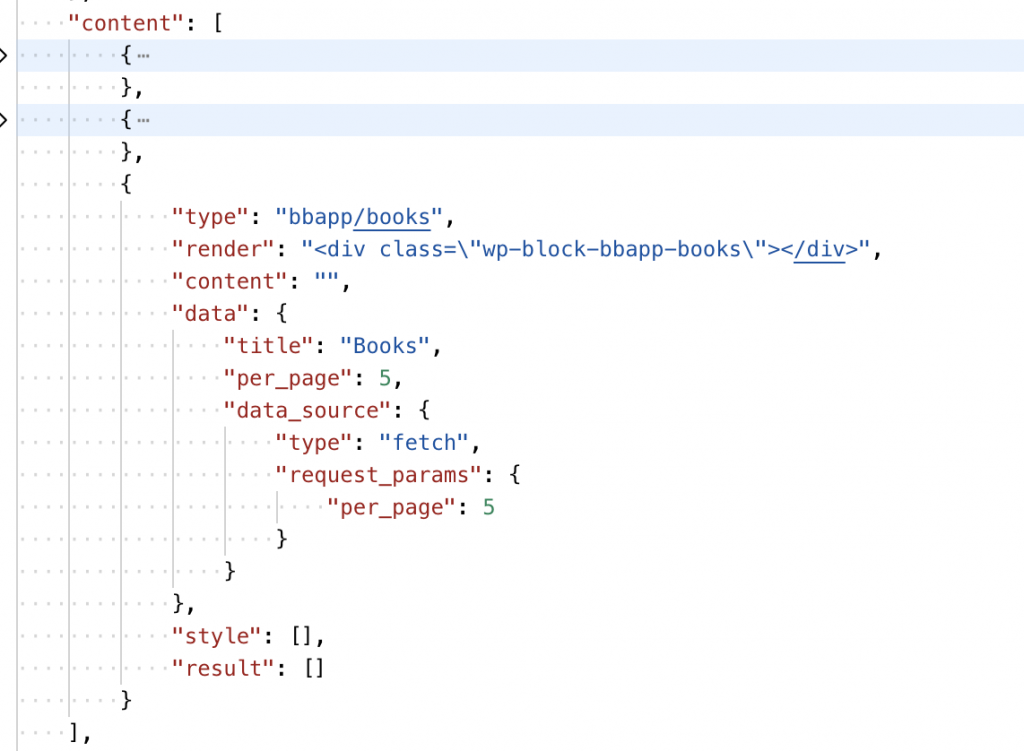
Template File:
use BuddyBossApp\Admin\GutenbergBlockAbstract; /** * Class Book * @package BuddyBossApp\Menus */ class ClasName extends GutenbergBlockAbstract { private static $instance; /** * Get the instance of the class. * * @return Book */ public static function instance() { if ( ! isset( self::$instance ) ) { $class = __CLASS__; self::$instance = new $class; } return self::$instance; } public function __construct() { $this->set_namespace( 'bbapp/<blockName>' ); $this->set_title( "<blockTItle>" ); $this->set_description( "<blockDescription>" ); $this->set_icon( '<blockIcon>' ); $this->set_keywords( array( '<blocKkeyword>' ) ); $attributes = $this->get_attributes(); $this->set_attributes( $attributes ); $preview = $this->get_preview(); $this->set_preview( $preview ); $this->init(); } public function init() { parent::init(); add_filter( 'bbapp_custom_block_data', array( $this, 'update_block_data' ), 10, 2 ); } function get_attributes() { return array(); } function get_preview( ) { return "<div>" . esc_html_e('Preview', 'text-domain') . "</div>"; } function get_results( $attributes ) { // TODO: Implement get_results() method. } function update_block_data( $app_page_data, $block_data ) { $data_source = array( 'type' => 'fetch', 'request_params' => array( 'per_page' => 5, ), ); $app_page_data['data']['data_source'] = $data_source; return $app_page_data; } }
Example Plugin
You can assemble the two PHP template files below into a folder, to create a plugin that will add a new “Books” block, which can be viewed when editing an App Page or using the App Editor.
Note: For this to actually work in your app or website, we are assuming you have also already registered a “book” custom post type in WordPress. Alternatively, you can adjust this code to use your own custom post type.
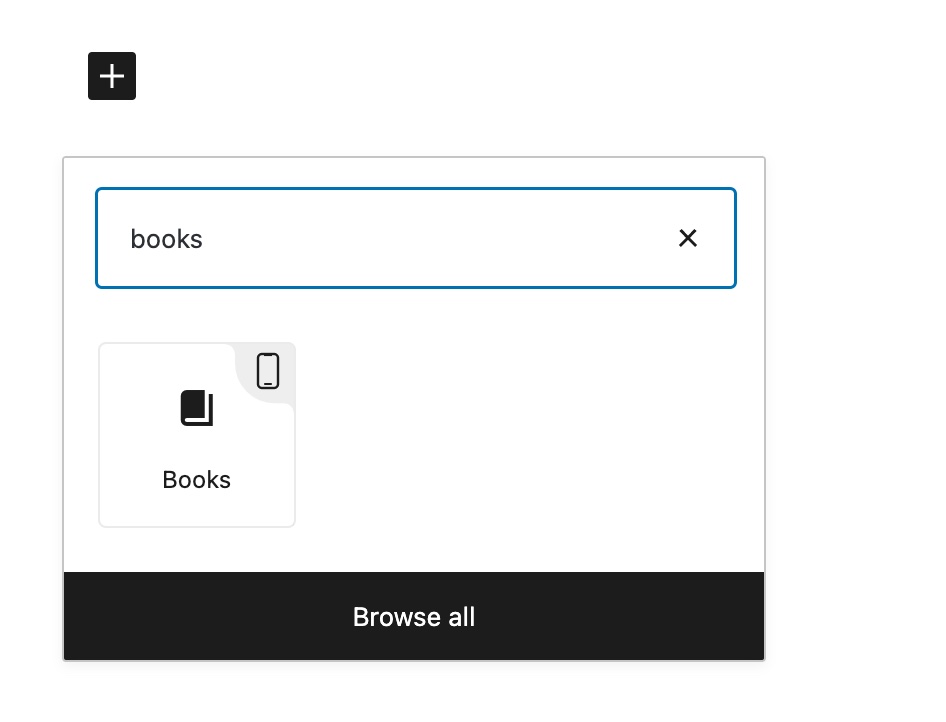
buddyboss-app-custom.php
This is the loader file. Create a plugin folder and add this file into it.
<?php /* Plugin Name: BuddyBoss App Custom Block Example Plugin URI: {add your own} Description: {add your own} Version: 1.0.0 Author: {add your own} Author URI: {add your own} */ if ( ! defined( 'ABSPATH' ) ) { exit(); } /** * Load Custom Block Functions */ function bbapp_custom_work_init() { if ( class_exists( 'bbapp' ) ) { include 'buddyboss-app-custom-block.php'; BuddyBossApp\Custom\BookBlock::instance(); } } add_action( 'plugins_loaded', 'bbapp_custom_work_init' );
buddyboss-app-custom-block.php
This file also goes into your plugin folder. This contains most of the functional code to register the new app block.
<?php namespace BuddyBossApp\Custom; use BuddyBossApp\Admin\GutenbergBlockAbstract; /** * Class Book * @package BuddyBossApp\Menus */ class BookBlock extends GutenbergBlockAbstract { private static $instance; /** * Get the instance of the class. * * @return Book */ public static function instance() { if ( ! isset( self::$instance ) ) { $class = __CLASS__; self::$instance = new $class; } return self::$instance; } public function __construct() { $this->set_namespace( 'bbapp/books' ); $this->set_title( "Books" ); $this->set_description( "Book" ); $this->set_icon( 'book' ); $this->set_keywords( array( 'book' ) ); $attributes = $this->get_attributes(); $this->set_attributes( $attributes ); $preview = $this->get_preview(); $this->set_preview( $preview ); $this->init(); } public function init() { parent::init(); add_filter( 'bbapp_custom_block_data', array( $this, 'update_block_data' ), 10, 2 ); } function get_attributes() { return array( array( 'name' => 'title', 'fieldtype' => 'text', 'default' => '', 'label' => 'Enter Title', ), array( 'name' => 'per_page', 'fieldtype' => 'number', 'default' => 5, 'label' => 'show items', ) ); } function get_preview( ) { return "<div> <h3>" . esc_html__('Books', '') . "</h3> <ul> <li id='li_one'> <div className='appboss_div'>" . esc_html__('Book Name', '') . "</div></li> <li id='li_two'> <div className='appboss_div' >" . esc_html__('Book Name', '') . "</div></li> <li id='li_three'> <div className='appboss_div' >" . esc_html__('Book Name', '') . "</div></li> </ul> </div>"; } function get_results( $attrs ) { return array(); } function update_block_data( $app_page_data, $block_data ) { $per_page = 5; // Per Page. if ( isset( $block_data['attrs']['per_page'] ) && ! empty( $block_data['attrs']['per_page'] ) ) { $per_page = absint( $block_data['attrs']['per_page'] ); } $data_source = array( 'type' => 'fetch', 'request_params' => array( 'per_page' => $per_page, ), ); $app_page_data['data']['data_source'] = $data_source; return $app_page_data; } }