Introduction
In this tutorial, you will learn how to add custom app screens to the Tab Bar and More Screen menus of the BuddyBoss App Plugin. For more information on how the Tab Bar works, see our Tab Bar tutorial.
If you are creating your own custom screens to be used in the app, you will need to add your screens into the menu system so that users will be able to navigate into your screens from within the app interface. You can optionally skip to the “Full Example Code” section at the end of the article, for an example plugin you can quickly test with.
Note: This tutorial requires you to create a new screen with the same name you creating a custom screen.
Note: This tutorial requires the BuddyBoss App Plugin v1.0.4 or higher.
Extending Class
To create a new custom screen inside the BuddyBoss App plugin, you need to extend the class called BuddyBossApp\Menus\Types\CoreAbstract
.
This class will contain some methods which you need to call & declare in order to register a new custom screen.
Method – setup()
Inside the class, we need to declare a setup method that is required. This function will contain all the code registering a new custom screen. This function is the init point where all methods and code should start from.
Usage Example
```php class BuddyBossAppBook extends CoreAbstract { public function __construct() { parent::__construct(); } public function setup() { // Calling a register screen method $this->register_screen( 'books', ...); //** ..and so on other code. // } ... } ```
Method – register_screen()
Use the register_screen
function to register a custom screen which is displayed at the tab bar section inside the BuddyBoss App plugin.
You can call this function multiple times to register multiple custom screens.
Parameters
- name – (Required | String) Name of the screen in lowercase containing not space and special characters.
- label – (Required | String) Label of the screen
- default_icon – (Required | String) Screen default icon name.
Tip – You can find icon name at/plugins/buddyboss-app/assets/app-icons/bbapp/*
- settings – (Optional | Array) Passing screen settings such as “deeplink_slug”.
- menu_metabox_callback – (Optional | Callback) Custom meta box for menu.
- menu_result_callback – (Optional | Callback ) Custom menu result callback.
- logged_in – (Optional | boolean ) default false. if provided true then screen will be not available for logged-out menu or tabbar.
Usage Example
```php class BuddyBossAppBook extends CoreAbstract { public function __construct() { parent::__construct(); } public function setup() { // Calling a register screen method $this->register_screen( 'books', __( 'Books', 'buddyboss-app' ), array( 'type' => 'buddyboss', 'id' => 'file' ) ); $this->register_screen( 'articles', __( 'Articles', 'buddyboss-app' ), 'bbapp/file', array(), null, null, true); // Menu not available to logged-out tabbar or more menu //.. and so on } ... } ```
The above code will appear like this:
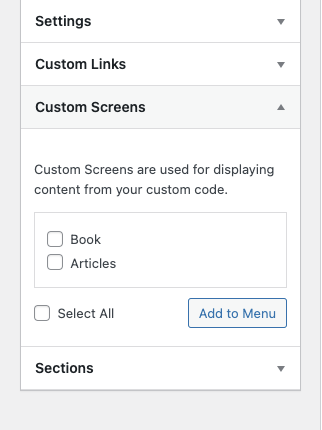
Method – register_screen_group()
Use this method to create a screen group. A single group can contain multiple screens. Once you register a screen with a group, that screen will appear separately in a group at the tab bar admin page.
Note: Calling this function is optional and it can be called only once.
Note: If this function is not called then all screens will appear inside the custom screen group by default.
Usage Example
```php class BuddyBossAppBook extends CoreAbstract { public function __construct() { parent::__construct(); } public function setup() { // Register Group $this->register_screen_group( 'reading_app_group', __( 'Reading App', 'buddyboss-app' ) ); // Calling a register screen method $this->register_screen( 'books', __( 'Books', 'buddyboss-app' ), array( 'type' => 'buddyboss', 'id' => 'file' ) ); $this->register_screen( 'articles', __( 'Articles', 'buddyboss-app' ), 'bbapp/file', array(), null, null, true); // Menu not available to logged-out tabbar or more menu //.. and so on. } ... } ```
The above code will appear like this:
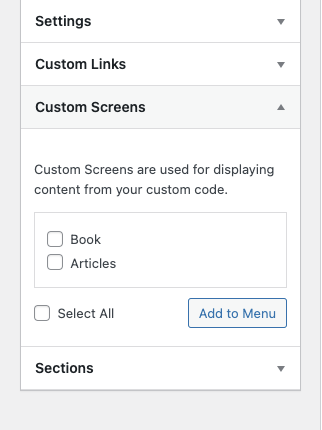
Full Example Code
```php class MyReadingApp extends CoreAbstract { public function __construct() { parent::__construct(); } public function setup() { // Register Group $this->register_screen_group( 'reading_app_group', __( 'Reading App', 'buddyboss-app' ) ); // Calling a register screen method $this->register_screens(); //.. and so on. } public function register_screens() { $this->register_screen( 'books', __( 'Books', 'buddyboss-app' ), 'bbapp/file' ); $this->register_screen( 'articles', __( 'Articles', 'buddyboss-app' ), 'bbapp/file', array(), null, null, true); // Menu not available to logged-out tabbar or more menu } } add_action( 'init', function () { MyReadingApp::instance(); } ); ```