In this tutorial, you will learn how to edit your theme templates when they are loaded as web fallbacks in the in-app browser. You can also target CSS when content is loaded in the in-app browser.
When content from your WordPress site is not supported natively in the BuddyBoss App, users will see a “web fallback”. This means they will see their content loaded in a web view within the app, displaying in an in-app browser. The content will use the styling from the active WordPress theme on your website, and will look the same as your responsive website looks when viewed from a mobile browser, but with some parts of the theme hidden depending on the scenario (explained below).
Note that there are two different types of web fallbacks, depending on the scenario. (1) WordPress Pages always display as a web fallback if used in the app. (2) Blog Posts and LearnDash will display their content natively, but only when supported Gutenberg blocks are being used. If any unsupported blocks are used, the content will open in a web fallback modal. Both scenarios are explained in the rest of this tutorial.
1. Web Fallbacks in WordPress Pages
If a WordPress Page is added to the Tab Bar or More Screen, and if the site is using BuddyBoss Theme, we automatically hide the header and footer of the theme, so the page fits in nicely within the app interface. For more information on how this works, see our video on Web Fallbacks.
You may want to add the functionality to remove the header and footer to a different WordPress theme, or edit the templates further, in which case you can use this function to check whether a template is being loaded in the in-app browser or not:
bbapp_is_loaded_from_inapp_browser
To disable this functionality entirely, you can use this function:
bbapp_disable_default_inapp_browser_template
Once you have checked that the theme is loading in the in-app browser, you can add code to hide the header, footer, and any other elements in that scenario. Depending on your theme, you may need to edit CSS as well to make the site look good with the header and footer removed. Make sure you still load all of your CSS and JavaScript in the site header and footer, otherwise your layout will break. Your goal should be to hide the header and footer visually but to keep the assets that WordPress normally loads.
Template File:
function remove_header_footer_for_buddyboss_app() { if ( function_exists( 'bbapp_is_loaded_from_inapp_browser' ) && bbapp_is_loaded_from_inapp_browser() ) { /* Disable the default template which loads on mobile app */ if ( function_exists( 'bbapp_disable_default_inapp_browser_template' ) ) { bbapp_disable_default_inapp_browser_template(); } /* Remove WP Adminbar */ add_filter( 'show_admin_bar', '__return_false', 99 ); /* Remove Theme Header Footer */ // Add and remove hook to remove your theme header and footer } } add_action( 'init', 'remove_header_footer_for_buddyboss_app' );
Example Implementation:
function bb_theme_remove_header_footer_for_buddyboss_app() { if ( function_exists( 'bbapp_is_loaded_from_inapp_browser' ) && bbapp_is_loaded_from_inapp_browser() ) { /* Disable the default template which loads on mobile app */ if ( function_exists( 'bbapp_disable_default_inapp_browser_template' ) ) { bbapp_disable_default_inapp_browser_template(); } /* Remove WP Adminbar */ add_filter( 'show_admin_bar', '__return_false', 99 ); /* Remove Theme Header Footer */ remove_action( THEME_HOOK_PREFIX . 'header', 'buddyboss_theme_header' ); remove_action( THEME_HOOK_PREFIX . 'header', 'buddyboss_theme_mobile_header' ); remove_action( THEME_HOOK_PREFIX . 'header', 'buddyboss_theme_header_search' ); remove_action( THEME_HOOK_PREFIX . 'footer', 'buddyboss_theme_footer_area' ); remove_action( THEME_HOOK_PREFIX . 'before_page', 'buddyboss_theme_buddypanel' ); /* Remove Header Class */ add_filter( 'body_class', function ( array $classes ) { if ( in_array( 'sticky-header', $classes ) ) { unset( $classes[ array_search( 'sticky-header', $classes ) ] ); } return $classes; } ); } } add_action( 'init', 'bb_theme_remove_header_footer_for_buddyboss_app' );
Testing in a Desktop Browser
While implementing the above code, it will be useful for you to test your changes in your desktop’s web browser, so you don’t have to deploy your code changes to your website and then view them in the app while developing. You can force the browser to render the WordPress page with the above code, the same way the app would render the content.
Keep in mind in the actual app, this content will be loaded with a native header and possibly a Tab Bar surrounding the content at the top and bottom. Also keep in mind this is specifically for WordPress pages loading in a web fallback and is not going to work for testing Blog Posts or LearnDash web fallbacks (next section in the tutorial).
To get started, add this extension to Chrome:
https://chrome.google.com/webstore/detail/modheader/idgpnmonknjnojddfkpgkljpfnnfcklj?hl=en
Make sure to enable the extension. Then while viewing a page in your WordPress site, click on the Extensions icon in Chrome, and click on “ModHeader”.
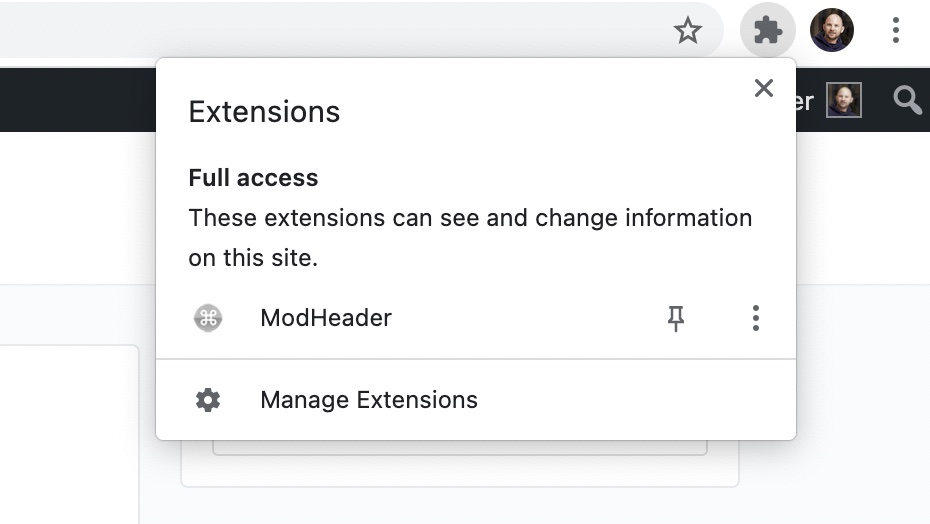
Then, in the ModHeader settings panel, for Request headers, enter ‘pagemode’ in the Name input, and ‘template’ in the Value input.
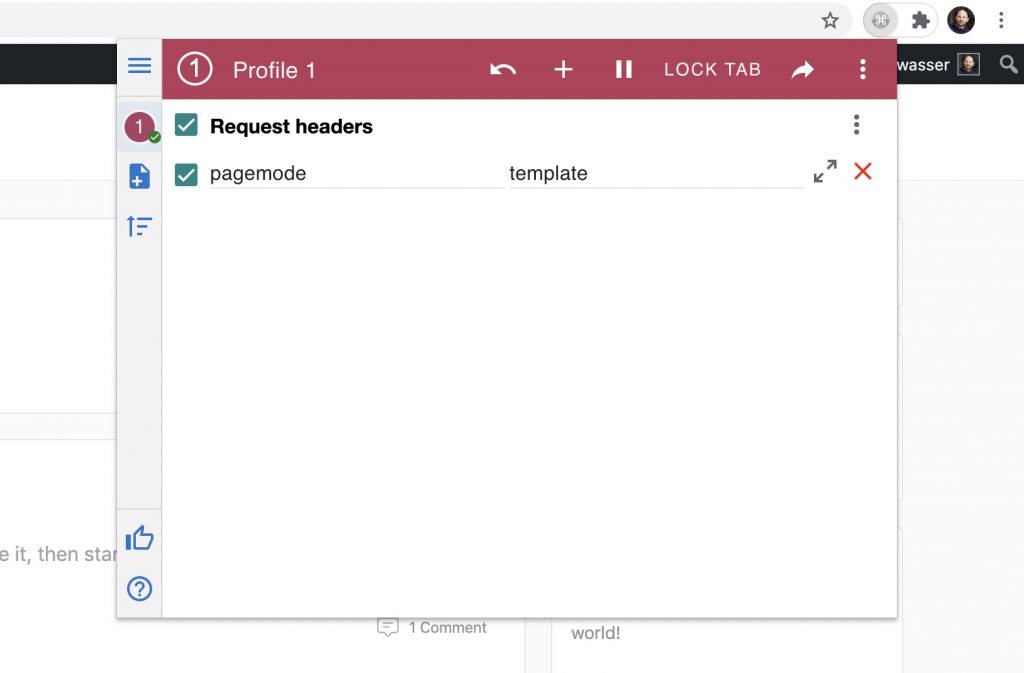
Now refresh the page. You should see your changes applied. If you are using the BuddyBoss Theme, you will see the theme’s header and footer removed, but all other content including the sidebar will still display.
To undo this, you will need to disable the ModHeader options, and then clear your browser’s cookies (or just remove the ‘bbapp_page_mode’ cookie), and then refresh the page.
2. Web Fallbacks in Blog Posts and LearnDash
If the content is added specifically into a Blog Post or LearnDash content, and if the content is using any unsupported blocks, then it will also display as a web fallback. In this scenario, the web content will display in a modal within the interface, and will only display what is coming from wp_content
with the rest of the theme hidden (header, footer, sidebar, etc).
This works automatically in BuddyBoss Theme and should work in other themes, but you may need to target CSS in certain themes to get the best-looking experience. For more information about web fallbacks coming from using unsupported blocks, see our video on Blog Posts (you can fast forward to 2:15 in the video).
Testing in a Desktop Browser
While writing code, it will be useful for you to test your changes in your desktop’s web browser, so you don’t have to deploy your code changes to your website and then view them in the app while developing. You can force the browser to render the Blog Post or LearnDash content the same way the app would render the content.
Keep in mind in the actual app, this content will be loaded within a scrollable modal. Also keep in mind this is specifically for Blog Posts and LearnDash content loading in a web fallback and is not going to work for testing WordPress Pages in a web fallback (previous section in the tutorial).
Go to any Blog Post or LearnDash course, lesson, or topic, and append the following to the end of the URL in your browser, and then refresh the page:
/?mobile-view-content=1
Example:
http://localhost/blog-post-name/?mobile-view-content=1
This will automatically remove the header, footer, sidebar, and all content outside of wp_content
. To undo this, just remove /?mobile-view-content=1
from the URL and refresh the page.
Targeting CSS in a Web Fallback
Whenever content is loading within an in-app browser, the BuddyBoss App plugin will add the following CSS class to the body
element on the page:
in-bbapp
By targeting this class, you can add CSS that will only display in your theme when the content is loaded within an in-app browser. Example:
body.in-bbapp {
background-color: red !important;
}
This will make the background red on the page, when viewed from an in-app browser.