In this tutorial, you will learn how to add more filters for segmenting which users will receive a notification that is sent manually from the Send Notification page of the BuddyBoss App Plugin. For more information on how push notifications work, see our Push Notifications tutorial.
You can optionally skip to the “Example Plugin” section at the end of the article, for an example plugin you can quickly test with.
To start, you will need to extend our abstract class by following these steps:
1. Create one class file and extend ‘BuddyBossApp\Notification\IntegrationAbstract’
use BuddyBossApp\UserSegment\SegmentsAbstract;
class <ClassName> extends SegmentsAbstract {
// Class code
}
2. Extend two abstract methods into the newly created class from Step 1.
use BuddyBossApp\UserSegment\SegmentsAbstract; class <ClassName> extends SegmentsAbstract { function filter_users( $user_ids ) { // TODO: Implement filter_users() method. } function render_script() { // TODO: Implement render_script() method. } }
3. Register a group for your push notifications.
name : Segment group.
label : Segment Group label.
show_child : Segment filter should show as a child filter or not.
$this->add_group( $group_name, $group_label, $show_child = false );
4. Once a group has been registered, add a segment filter into the created group using the below method:
group_name : Segment group type.
filter_name : Segment filter name.
fields : Segment filter field name to select filter items. To create a field, refer to the next step.
args : Custom argument. You need to send a `label` parameter for the custom filter.
$this->add_filter( $group_name, $filter_name, $fields = array(), $args = array() );
5. Create a Segment filter field by using this method:
field_name : Segment filter field name.
Field_type : Segment filter field type. Example: Checkbox.
args : field arguments
Options : Filter options
Multiple : Select multiple or single
Empty_message : Message for empty filter values.
$this->add_field( $field_name, $field_type, $args = array() );
6. Allow filter to get user ID by ‘filter_users‘ method.
function filter_users( $user_ids ) { $filter = $this->get_filter_data_value( 'filter' ); $selected_item = (array) $this->get_filter_data_value( $field_name ); $_user_ids = array(); switch ( $filter ) { case '{$group_name}_{$filter_name}': // Find Userid using selected item. break; } if ( ! empty( $_user_ids ) ) { return array_merge( $user_ids, $_user_ids ); } return $user_ids; }
Template File:
<?php /** * Register custom Segment for manual notification. */ namespace BuddyBossApp\Custom; use BuddyBossApp\UserSegment\SegmentsAbstract; class ClassName extends SegmentsAbstract { /** * BookSegment constructor. */ public function __construct() { $this->add_group( $group_name, $group_label, $show_child = false ); $this->add_filter( $group_name, $filter_name, $fields = array(), $args = array() ); $this->add_field( $field_name, $field_type, array( "options" => $options, "multiple" => true, "empty_message" => __( "No items found.", "buddyboss-app" ), ) ); } function filter_users( $user_ids ) { $filter = $this->get_filter_data_value( 'filter' ); $selected_item = (array) $this->get_filter_data_value( $field_name ); $_user_ids = array(); switch ( $filter ) { case '{$group_name}_{$filter_name}': // Find Userid using selected item. break; } if ( ! empty( $_user_ids ) ) { return array_merge( $user_ids, $_user_ids ); } return $user_ids; } function render_script() { // TODO: Implement render_script() method. } }
Example Plugin
You can assemble the two PHP template files below into a folder, to create a plugin that will add a new “Books” segment in the Push Notifications form at BuddyBoss App > Push Notifications > Send New.
Note: For this to actually work in your app, we are assuming you have also already registered a “book” custom post type in WordPress. Alternatively, you can adjust this code to use your own custom post type.
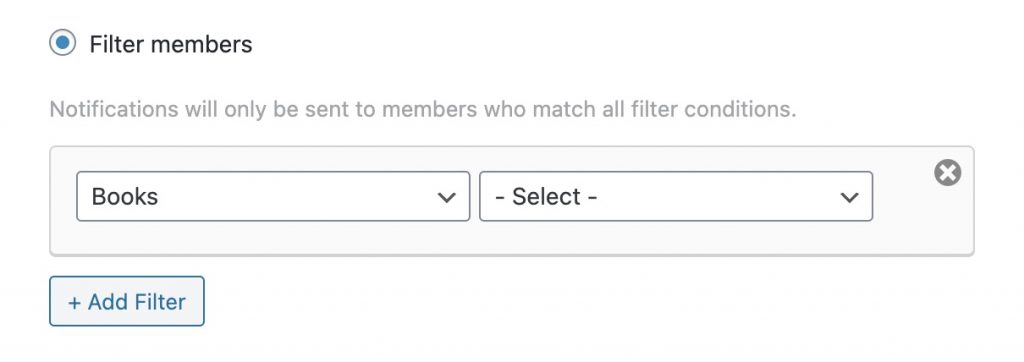
buddyboss-app-custom.php
This is the loader file. Create a plugin folder and add this file into it.
<?php /* Plugin Name: BuddyBoss App Custom Push Notification Example Plugin URI: {add your own} Description: {add your own} Version: 1.0.0 Author: {add your own} Author URI: {add your own} */ if ( ! defined( 'ABSPATH' ) ) { exit(); } /** * Load Custom Push Notification */ function bbapp_custom_work_init() { if ( class_exists( 'bbapp' ) ) { include 'buddyboss-app-custom-manual-push-notification.php'; new BuddyBossApp\Custom\BookSegment(); } } add_action( 'plugins_loaded', 'bbapp_custom_work_init' );
buddyboss-app-custom-manual-push-notification.php
This file also goes into your plugin folder. This contains most of the functional code to register the new push notification filter.
<?php /** * Register custom Segment for manual notification. */ namespace BuddyBossApp\Custom; use BuddyBossApp\UserSegment\SegmentsAbstract; /** * Class Book * @package BuddyBossApp\Menus */ class BookSegment extends SegmentsAbstract { /** * BookSegment constructor. */ public function __construct() { $this->add_group( 'books', __( "Books", "buddyboss-app" ) ); $this->add_filter( "books", "user_books", array( "book_select" ), array( 'label' => __( 'Book Authors', 'buddyboss-app' ), ) ); $book_options = array(); $book_query = new \WP_Query( array( 'post_type' => 'book ', 'fields' => 'ids', 'nopaging' => true, 'orderby' => 'name', 'order' => 'asc', 'post_status' => array( 'publish' ), ) ); if ( $book_query->have_posts() ) { foreach ( $book_query->posts as $book_id ) { $book_options[ $book_id ] = get_the_title( $book_id ); } } $this->add_field( "book_select", "Checkbox", array( "options" => $book_options, "multiple" => true, "empty_message" => __( "No books found.", "buddyboss-app" ), ) ); $this->load(); } /** * @param $user_ids * * @return array */ function filter_users( $user_ids ) { $filter = $this->get_filter_data_value( 'filter' ); $book_ids = (array) $this->get_filter_data_value( 'book_select' ); $_user_ids = array(); switch ( $filter ) { case 'books_user_books': // Write logic to get user ids based on selected value. // Here I'm simply return books author foreach ( $book_ids as $book_id ) { $_user_ids[] = get_post_field( 'post_author', $book_id ); } } if ( ! empty( $_user_ids ) ) { return array_merge( $user_ids, $_user_ids ); } return $user_ids; } /** * */ function render_script() { // TODO: Implement render_script() method. } }