If you haven’t done any custom development on the app before, please first follow this tutorial.
Updating your custom development repo:
BuddyBoss App now supports native libraries, which allows you to add and configure native libraries and write your own native modules inside your custom development repo.
Your custom development repo will need to be updated to our new project structure in order to continue doing custom development with BuddyBoss App, and to add support for using native libraries. Follow the instructions to auto-update the project in your repo.
- If on a Mac, open Terminal. If on Windows, open your Command Prompt.
- Make sure you have the latest version of BuddyBoss Bundler by running this command.
npm install -g buddyboss-bundler
- In the command line, navigate (cd) into your Git repo.
cd ./your-project
- In the command line Enter
buddy boss-bundler upgrade
command. - Follow the instruction in the command line to complete the upgrade.
Running this script inside your repo will try to install all missing project files required for doing custom development with BuddyBoss App.
Once the update process is done commit the changes into the Git server and then request a new app build. Once the build is complete, download the build to your device, and then go back into the command line to start a new session in BuddyBoss Bundler.
You should now be able to continue doing custom development, and any native libraries you added should be usable in the app. If you try to add new native libraries after, you will need to generate new builds again before they can be used in your test app.
Installing native libraries
You can already install npm packages that don’t contain native code (JavaScript libraries) with a simple npm install. But to install libraries that are using native code, use the following command instead of installing with npm.
Installing
buddyboss-bundler install <package>
For the specific npm package:
buddyboss-bundler install <package>@<package>
Uninstalling
buddyboss-bundler uninstall <package>
For the specific npm package:
buddyboss-bundler uninstall <package>@<package>
Note: BuddyBoss Bundler Install command will show a warning about rebuilding the app when you install a native package.
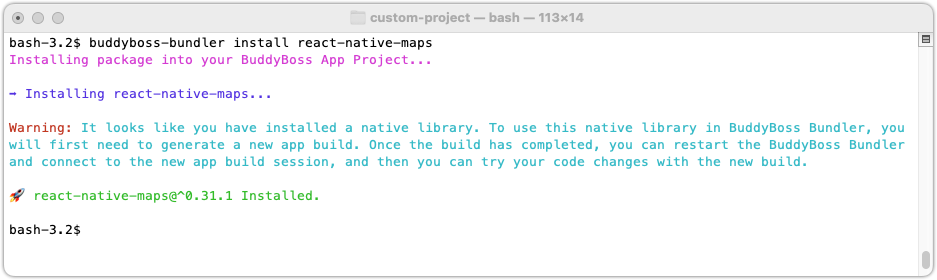
Why is this needed?
Packages with native code must be linked to the core app’s native project during the build process. In order for React Native’s auto-linking to work as intended, these native packages must be installed into the main project’s package.json. The command above will add the package to the nativeDependencies
and podDependecies
of package.json, which instructs the build server to install the package on the core app project when you generate the next app build. Buddyboss Bundler Install automatically recognises the native module npm package and configures it with the project.
Note: Packages with native code require you make a new build before you can use them from your code, unlike the packages that you install with npm, which can be used immediately.
Configuring native packages
If your package does not require initialization on the native side (most do not), after generating a new app build, you can import and use your package as described in your package’s documentation.
If your package requires initialization through writing native code you can use the methods inside the iOS and Android projects included in your custom development repo. These methods hook into the native methods inside the BuddyBoss core app’s native projects allowing you to use them as you would during regular React Native development.
Let’s say you want to install the Google Maps plugin (https://github.com/react-native-maps/react-native-maps).
The first thing to do is to install the package with:
buddyboss-bundler install react-native-maps
Since per documentation of the package, it requires initialization, instead of changing AppDelegate.m
file on iOS, and android/app/src/main/AndroidManifest.xml
file on Android you would change the corresponding files of our BuddybossCustomCode module, like this
iOS:
File: ios/BuddybossCustomCode.m |
#import "BuddybossCustomCode.h" #import <React/RCTRootView.h> #import <GoogleMaps/GoogleMaps.h> @implementation BuddybossCustomCode // Lifecycle methods (DO NOT DELETE) // These methods will be called in the BuddyBoss app's AppDelegate.m // You can hook into them to initiate your native libraries or run any custom side-effects // Called inside AppDelegate.m didFinishLaunchingWithOptions method. This should be used to init most native libraries. + (void)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions { [GMSServices provideAPIKey:@"YOUR_GOOGLE_API_KEY"]; // add this line using the api key obtained from Google Console } // Called inside AppDelegate.m didFinishLaunchingWithOptions method when rootView is attached to window + (void)rootViewVisible:(RCTRootView *)rootView {} …
Android:
File: android/app/src/main/AndroidManifest.xml |
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.buddybosscustomcode">
<application>
<meta-data
android:name="com.google.android.geo.API_KEY"
android:value="YOUR_GOOGLE_API_KEY"/>
<!-- You will also only need to add this uses-library tag -->
<uses-library android:name="org.apache.http.legacy" android:required="false"/>
</application>
</manifest>
You can skip the step “Configure Google Play Services” from their documentation, as this is already included in the core app.
What is needed is to generate a new app build, and then run buddyboss-bundler
to start using the package.
Note: If your package requires a native method that is not exposed please contact our support team and we will try to include it in the project.